하아찡
[C#/WPF] Login 프로젝트 SingUp 본문
회원가입 창입니다.
현재는 데이터를 이름, 아이디, 비밀번호, 이메일만 받는 형식으로 작업했으며 나중에 데이터가 추가될 예정입니다.
작업결과물
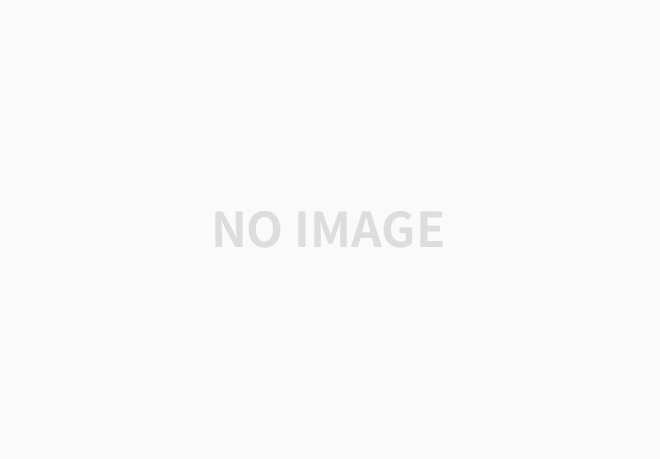
SingUp.xaml
<UserControl x:Class="CoinLogin.Views.SingUp"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/"
xmlns:tp="clr-namespace:TextBoxPlaceHolder;assembly=TextBoxPlaceHolder"
Width="350"
prism:ViewModelLocator.AutoWireViewModel="True">
<UserControl.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="pack://application:,,,/TextBoxplaceHolder;component/RTextBoxPlaceHolder.xaml" />
<ResourceDictionary Source="pack://application:,,,/TextBoxplaceHolder;component/RPasswordPlaceHolder.xaml" />
<ResourceDictionary Source="pack://application:,,,/CoinLogin;component/MyResource.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</UserControl.Resources>
<Border CornerRadius="15" BorderThickness="0" BorderBrush="Black" >
<Border CornerRadius="10" BorderThickness="6">
<Grid x:Name="MainGrid" >
<Grid.RowDefinitions>
<RowDefinition Height="1*" />
</Grid.RowDefinitions>
<StackPanel VerticalAlignment="Center" Orientation="Vertical">
<TextBlock Text="회원가입" Style="{StaticResource logintitleText}" Margin="0 0 0 20"/>
<tp:CTextBoxPlaceHolder Margin="0 0 0 10" Style="{StaticResource ResourceKey=TextBoxPlaceHolder}" HorizontalAlignment="Stretch" VerticalAlignment="Bottom" Height="50" TextValue="{Binding Name,Mode=TwoWay}" PlaceHolder="NAME"/>
<tp:CTextBoxPlaceHolder Margin="0 0 0 10" Style="{StaticResource ResourceKey=TextBoxPlaceHolder}" HorizontalAlignment="Stretch" VerticalAlignment="Bottom" Height="50" TextValue="{Binding ID,Mode=TwoWay}" PlaceHolder="ID"/>
<tp:CPasswordBoxPlaceHolder Margin="0 0 0 10" Style="{StaticResource ResourceKey=PasswordPlaceHolder}" HorizontalAlignment="Stretch" VerticalAlignment="Bottom" Height="50" TextValue="{Binding PW,Mode=TwoWay}" PlaceHolder="PW"/>
<tp:CPasswordBoxPlaceHolder Margin="0 0 0 10" Style="{StaticResource ResourceKey=PasswordPlaceHolder}" HorizontalAlignment="Stretch" VerticalAlignment="Bottom" Height="50" TextValue="{Binding RePW,Mode=TwoWay}" PlaceHolder="RE-PW"/>
<tp:CTextBoxPlaceHolder Margin="0 0 0 10" Style="{StaticResource ResourceKey=TextBoxPlaceHolder}" HorizontalAlignment="Stretch" VerticalAlignment="Bottom" Height="50" TextValue="{Binding Email,Mode=TwoWay}" PlaceHolder="EMAIL"/>
<Button Margin="0 10 0 10" Style="{StaticResource ResourceKey=loginbutton}" Grid.Column="1" Command="{Binding CommandSinUp}" Content="회원가입" />
<WrapPanel Margin="5" Grid.Column="0" HorizontalAlignment="Right" Orientation="Horizontal" VerticalAlignment="Bottom">
<Button Style="{StaticResource ResourceKey=btnsub}" Command="{Binding CommandNavigationBack}" Content="뒤로가기"></Button>
</WrapPanel>
</StackPanel>
</Grid>
</Border>
</Border>
</UserControl>
SingUpViewModel.cs
using DB;
using Prism.Commands;
using Prism.Mvvm;
using Prism.Regions;
using System;
using System.Windows;
namespace CoinLogin.ViewModels
{
public class SingUpViewModel : BindableBase
{
#region Prism
private IRegionManager _rm;
#endregion
#region Model
private string name;
public string Name
{
get { return name; }
set { SetProperty(ref name, value); }
}
private string id;
public string ID
{
get { return id; }
set { SetProperty(ref id, value); }
}
private string pw;
public string PW
{
get { return pw; }
set { SetProperty(ref pw, value); }
}
private string repw;
public string RePW
{
get { return repw; }
set { SetProperty(ref repw, value); }
}
private string eamil;
public string Email
{
get { return eamil; }
set { SetProperty(ref eamil, value); }
}
#endregion
#region Event
private DelegateCommand commandnavigationback;
public DelegateCommand CommandNavigationBack =>
commandnavigationback ?? (commandnavigationback = new DelegateCommand(ExecuteCommandNavigationBack));
void ExecuteCommandNavigationBack()
{
_rm.RequestNavigate("LoginView", "Login");
}
private DelegateCommand commandsingup;
public DelegateCommand CommandSinUp =>
commandsingup ?? (commandsingup = new DelegateCommand(ExecuteCommandSinUp));
void ExecuteCommandSinUp()
{
if (Mssql.Instance.GetConnection() == false)
{
MessageBox.Show("서버가 닫혀있습니다. 비회원으로 이용해주세요.");
ExecuteCommandNavigationBack();
}
string message = "";
if (Name == "")
message = "이름을 입력해주세요.";
else if (ID == "")
message = "아이디를 입력해주세요.";
else if (PW == "" || RePW == "")
message = "비밀번호를 입력해주세요.";
else if (PW != RePW)
message = "입력하신 비밀번호가 서로 다릅니다.";
if (message != "")
{
MessageBox.Show(message);
return;
}
string Query = $"SELECT * FROM ACCOUNT WHERE ID = '{ID}'";
try
{
bool IDDuplication = Mssql.Instance.HasRows(Query);
if (IDDuplication)
{
message = "아이디 중복입니다.";
MessageBox.Show(message);
return;
}
Query = $"INSERT INTO ACCOUNT(ID, PW, USERNAME, EMAIL,USEMARKET) VALUES ('{ID}', '{PW}','{Name}' ,'{Email}', 0)";
Mssql.Instance.ExecuteNonQuery(Query);
MessageBox.Show("회원가입 완료");
ExecuteCommandNavigationBack();
}
catch(Exception ex)
{
MessageBox.Show("ExecuteCommandSinUp DB오류");
ExecuteCommandNavigationBack();
}
}
#endregion
public SingUpViewModel(IRegionManager rm)
{
_rm = rm;
PW = "";
RePW = "";
}
}
}
반응형
'C# > 코인프로그램 - 코드' 카테고리의 다른 글
[C#/WPF] LoginDB (0) | 2023.12.12 |
---|---|
[C#/WPF] Login 프로젝트 Login (0) | 2023.12.12 |
[C#/WPF] Login 프로젝트 LoginMain (0) | 2023.12.12 |
[C#/WPF/수정] Upbit 프로젝트 Chart 매수 매도 선 추가 (1) | 2023.12.08 |
[C#/WPF/수정] Upbit프로젝트 Balance 타이머 추가 (1) | 2023.12.08 |